Peter age is 10
All Tutorials Links
Design Patterns
Servlets
JDBC
JAVA
Spring & Spring Boot & WebService & Database & AWS
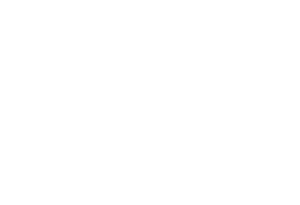
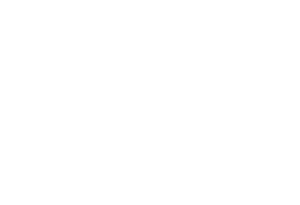
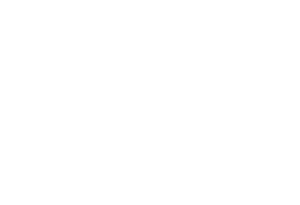
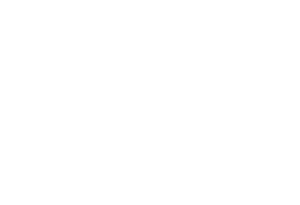
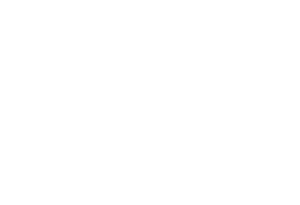
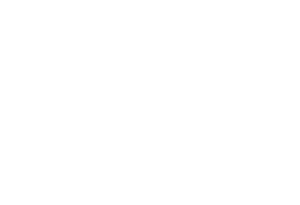
- All JavaEE Viedos Playlist
- All JavaEE Viedos
- All JAVA EE Links
- All Design Patterns Links
- Spring Tutorial
- Spring boot & JMS & Apache Kafka & Web Services
- Servlets Tutorial
- JDBC Tutorial
- JAVA Tutorial
- Java Collection Framework
- Apache Server Tutorial
- Apache Maven Tutorial
- JSON/XML/SQL/MongoDB Tutorials
- Computer Tutorial
- Kids Learning Tutorial
- Cooking Tutorial
- All Design Patterns Links
- Design Pattern - Playlists
- Design Pattern - Playlists - Part1
- Design Patterns - Introduction - Playlist
- J2EE Design Patterns - Playlist
- Creational Design patterns - Playlist
- Structural Design patterns - Playlist
- Behavioral Design patterns - Playlist
- Front Controller Design Pattern - Playlist
- Intercepting Filter Design Pattern - Playlist
- Business Delegate Design Pattern - Playlist
- Service Locator Design Pattern - Playlist
- Context Object Design Pattern - Playlist
- Data Access Object Design Pattern - Playlist
- Design Pattern - Playlists - Part2
- Object Pool Design Pattern - Playlist
- Singleton Design Pattern - Playlist
- Factory Design Pattern - Playlist
- Abstract Factory Design Pattern - Playlist
- Prototype Design pattern - Playlist
- Builder Design pattern - Playlist
- Iterator Design Pattern - Playlist
- Observer Design Pattern - Playlist
- Chain of Responsibility Design Pattern - Playlist
- Memento Design pattern - Playlist
- Design Pattern - Playlists - Part3
- Mediator Design pattern - Playlist
- Strategy Design pattern - Playlist
- Visitor Design pattern - Playlist
- State Design pattern - Playlist
- Command Design pattern - Playlist
- Interpreter Design pattern - Playlist
- Filter or Criteria Design Pattern - Playlist
- Composite Design Pattern - Playlist
- Proxy Design pattern - Playlist
- Flyweight Design pattern - Playlist
- Decorator Design pattern - Playlist
- Bridge Design pattern - Playlist
- Adapter Design pattern - Playlist
- Design Pattern - Playlists - Part4
- Base Design Pattern - Playlist
- Data Source Architectural Design Patterns - Playlist
- Object-Relational Metadata Mapping Design Patterns - Playlist
- Offline Concurrency Design Patterns - Playlist
- Domain Logic Design Patterns - Playlist
- Object-Relational Behavioral Design Patterns - Playlist
- Object-Relational Structural Design Patterns - Playlist
- Web Presentation Design Patterns - Playlist
- Distribution Design Patterns - Playlist
- Session State Design Patterns - Playlist
- Concurrency Design patterns - Playlist
- Design Pattern - Playlists - Part5
- Design Pattern - Playlists - Part1
- Design Patterns - Introduction
- Design Patterns
- Design patterns - catalog
- Enterprise Application Architecture Patterns
- Domain Logic Patterns
- Data Source Architectural Patterns
- Object Relational Behavioral Patterns
- Object-Relational Structural Patterns
- Object Relational Metadata Mapping Patterns
- Web Presentation Patterns
- Distribution Patterns
- Offline Concurrency Patterns
- Base Design Pattern
- Session State Design pattern
- Concurrency patterns
- J2EE patterns
- Creational Design patterns
- Structural design patterns
- Behavioral design patterns
- J2EE Design Patterns
- Creational Design patterns
- Creational Design patterns
- Object Pool Design Pattern
- Singleton Design Pattern
- Factory Design Pattern
- Abstract Factory Design Pattern
- Prototype Design Pattern
- Builder Design Pattern
- Builder Design Pattern - Introduction
- Builder Design pattern - Real Time Example [Meal Package]
- Builder Design pattern - Real Time Example [Animal Toys]
- Builder Design pattern - Real Time Example [Beverage]
- Builder Design Pattern - Class Diagram
- Builder Design Pattern - Sequence Diagram
- Builder Design Pattern - Implementation [Beverage]
- Builder Design Pattern - Implementation [Animal Toy]
- Builder Design Pattern - KeyPoints
- Behavioral design patterns
- Behavioral design patterns
- Iterator Design Pattern
- Observer Design Pattern
- Chain of Responsibility Design Pattern
- Chain of Responsibility Design Pattern - Introduction
- Chain of Responsibility Design Pattern - Real time Example
- Chain of Responsibility Design Pattern - Class and Sequence Diagram
- Chain of Responsibility Design Pattern - Implementation(One Receiver)
- Chain of Responsibility Design Pattern - Implementation(one or more Receiver)
- Chain of Responsibility Design Pattern - Key Points
- Momento Design pattern
- Template Design pattern or Template Method Design pattern
- Template Design pattern or Template Method Design pattern - Introduction
- Template Design pattern or Template Method Design pattern - Real Time Example [Coffee]
- Template Design pattern or Template Method Design pattern - Real Time Example [Car]
- Template Design pattern or Template Method Design pattern - Class Diagram
- Template Design pattern or Template Method Design pattern - Implementation [Building a House]
- Template Design pattern or Template Method Design pattern - Implementation [Coffee]
- Template Design pattern or Template Method Design pattern - KeyPoints
- Mediator Design pattern
- Mediator Design pattern - Introduction
- Mediator Design pattern - When to Use
- Mediator Design pattern - Real Time Example [Facebook]
- Mediator Design pattern - Real Time Example [ATC]
- Mediator Design pattern - Real Time Example [Chat Room]
- Mediator Design pattern - Class Diagram
- Mediator Design pattern - Implementation [Facebook]
- Mediator Design pattern - Implementation [Chat Room]
- Mediator Design pattern - KeyPoints
- Strategy Design pattern
- Strategy Design pattern - Introduction
- Strategy Design pattern - Real Time Example [Compress files]
- Strategy Design pattern - Real Time Example [Payment]
- Strategy Design pattern - Real Time Example [Travel]
- Strategy Design pattern - Real Time Example [Sorting]
- Strategy Design pattern - Real Time Example [Search]
- Strategy Design pattern - Class Diagram
- Strategy Design pattern - Sequence Diagram
- Strategy Design pattern - Implementation [Compress files]
- Strategy Design pattern - Implementation [Travel]
- Strategy Design pattern - Implementation [Payment]
- Strategy Design pattern - Implementation [Search]
- Strategy Design pattern - Implementation [Sort]
- Strategy Design pattern - KeyPoints
- Visitor Design pattern
- Visitor Design pattern - Introduction
- Visitor Design pattern - Real time Example [Kids]
- Visitor Design pattern - Real time Example [Products]
- Visitor Design pattern - Class Diagram
- Visitor Design pattern - Implementation [Kids]
- Visitor Design pattern - Implementation [Products]
- Visitor Design pattern - KeyPoints
- State Design pattern
- State Design pattern - Introduction
- State Design pattern - Real time example [ATM]
- State Design pattern - Real time example [TV Remote]
- State Design pattern - Real time example [Vending Machine]
- State Design pattern - Real time example [Project]
- State Design pattern - Class Diagram
- State Design pattern - Implementation [ATM]
- State Design pattern - Implementation [Vending Machine]
- State Design pattern - Implementation [Project]
- State Design pattern - Implementation [LED TV]
- State Design pattern - Keypoints
- Command Design pattern
- Command Design pattern - Introduction
- Command Design pattern - Real time example [Hotel]
- Command Design pattern - Real time example [Menu]
- Command Design pattern - Class Diagram
- Command Design pattern - Sequence Diagram
- Command Design pattern - Object Creation and flow
- Command Design pattern - Implementation [Menu]
- Command Design pattern - Key points
- Interpreter Design pattern
- Structural design patterns
- Structural design patterns
- Filter or Criteria Design Pattern
- Composite Design Pattern
- Proxy Design Pattern
- Proxy Design Pattern - Introduction
- Proxy Design pattern - Real Time Example[ATM]
- Proxy Design pattern - Real Time Example [Proxy Server]
- Proxy Design pattern - Class Diagram
- Proxy Design pattern - Sequence Diagram
- Proxy Design pattern - Implementation [Protection Proxy]
- Proxy Design pattern - Implementation [Remote Proxy]
- Proxy Design pattern - Implementation[Virtual Proxy]
- Proxy Design Pattern - Key Points
- Flyweight Design pattern
- Decorator Design pattern
- Decorator Design pattern - Introduction
- Decorator Design pattern - Real Time Example [Dosa]
- Decorator Design pattern - Real Time Example [Ice Cream]
- Decorator Design pattern - Real Time Example [Pizza]
- Decorator Design pattern - Real Time Example [Car]
- Decorator Design pattern - Class Diagram
- Decorator Design pattern - Sequence Diagram
- Decorator Design pattern - Implementation [Dosa]
- Decorator Design pattern - Implementation [Pizza]
- Decorator Design pattern - Implementation [Ice Cream]
- Decorator Design pattern - Implementation [Car]
- Decorator Design pattern - Implementation [Shape]
- Decorator Design pattern - Key Points
- Bridge Design pattern
- Bridge Design pattern - Introduction
- Bridge Design pattern - Real time example [Send Message]
- Bridge Design pattern - Real time example [Publish Message]
- Bridge Design pattern - Real time example [Shape]
- Bridge Design pattern - Real time example [TV]
- Bridge Design pattern - Class Diagram
- Bridge Design pattern - Implementation [Shape]
- Bridge Design pattern - Implementation [Send Message]
- Bridge Design pattern - Implementation [Publish Message]
- Bridge Design pattern - Implementation [LED TV]
- Bridge Design pattern - Keypoints
- Adapter Design Pattern
- Adapter Design pattern - Introduction
- Adapter Design Pattern - Real Time Exmaple(Translator)
- Adapter Design pattern - Real Time Example (Card Reader)
- Adapter Design pattern - Real Time Example (Mobile Charger)
- Adapter Design pattern - Real Time Example (Universal Adaptor)
- Adapter Design pattern - Real Time Example [JDBC Driver]
- Adapter Design pattern - Class diagram
- Adapter Design Pattern - Sequence diagram
- Adapter Design Pattern - Implementation [Language Translator]
- Adapter Pattern - Implementation [Object Adapter]
- Adapter Pattern - Implementation [Class Adapter]
- Adapter Design Pattern - Implementation [Mobile Charger]
- Adapter Design Pattern - Key Points
- Concurrency Design patterns
- Concurrency patterns
- Thread Pool Design Pattern
- Monitor or synchronization Design Pattern
- Lock Design Pattern
- Scheduler Design Pattern
- Read-write lock Design Pattern
- Messaging Design Pattern(MDP)
- Messaging Design Pattern(MDP) - Introduction
- Messaging Design Pattern(MDP) - Asynchronous messaging
- Messaging Design Pattern(MDP) - Two Way Messaging
- Messaging Design Pattern(MDP) - Implementation of Proxy
- Messaging Design Pattern(MDP)-Implementation of Adapter
- Messaging Design Pattern(MDP) - Implementation of Webservice
- Reactor Design Pattern
- Double-checked locking Design Pattern
- Offline Concurrency Patterns
- Session State Design pattern
- Distribution Patterns
- Base Design Pattern
- Base Design Pattern
- Gateway Design Pattern
- Layer Supertype Design Pattern
- Registry Design Pattern
- Money Design Pattern
- Plugin Design Pattern
- Special Case Design Pattern
- Separated Interface Design Pattern
- Service Stub Design Pattern
- Record Set Design Pattern
- Mapper Design Pattern
- Value Object Design Pattern
- Web Presentation Patterns
- Domain Logic Patterns
- Object Relational Structural Patterns
- Object-Relational Structural Patterns
- Identity Field Design Pattern
- Foreign Key Mapping Design Pattern
- Association Table Mapping Design Pattern
- Dependent Mapping Design Pattern
- Embedded Value Design Pattern
- Serialized LOB Design Pattern
- Single Table Inheritance Design Pattern
- Class Table Inheritance Design Pattern
- Concrete Table Inheritance Design Pattern
- Inheritance Mappers Design Pattern
- Object Relational Behavioral Patterns
- Object Relational MetadataMapping Patterns
- Data Source Architectural Patterns
- All Design Patterns Links
- Servlets Tutorial
- Servlets Tutorial - Playlists [Part 1]
- Servlets Tutorial - Playlist
- Tomcat - Playlist
- Servlets : WAR file - Playlist
- HTTP Methods and HTTP Status Codes - Playlist
- Client-Server Model - Playlist
- Servlet Application Creation using Eclipse - Playlist
- Servlets Basics - Playlist
- Servlets : Request and Response Headers - Playlist
- Servlets Form Data - Playlist
- Servlets : ServletConfig and ServletContext - Playlist
- Servlets : load-on-startup - Playlist
- Servlets : RequestDispatcher - Playlist
- Servlets : Send Redirect - Playlist
- Servlets Tutorial - Playlists [Part 2]
- Servlet Filter - Playlist
- Servlets : Filter Config - Playlist
- Servlets : HttpSession - Playlist
- Servlets : Cookies - Playlist
- Servlets : Attribute - Playlist
- Servlets : ServletRequestAttributeListener - Playlist
- Servlets : Listeners - Playlist
- Servlets : HttpSessionAttributeListener - Playlist
- Servlets : ServletContextAttributeListener - Playlist
- Servlets : ServletContextListener - Playlist
- Servlets : Upload File and Download File - Playlist
- Servlets : HttpSessionListener - Playlist
- Servlets : ServletRequestListener - Playlist
- Servlets : HttpSessionBindinglistener - Playlist
- Servlets : Session Tracking - Playlist
- Servlets : URL Rewriting - Playlist
- Servlets - Database Access - Playlist
- Servlets Basics - Part1
- Servlets Basics - Part2
- Servlets : Request and Response Headers
- Servlets Form Data
- ServletConfig and ServletContext
- Servlets : load-on-startup
- RequestDispatcher and Send Redirect
- RequestDispatcher Introduction
- Servlets : RequestDispatcher Example
- Servlets : Send Redirect Introduction
- Send Redirect Demo[Transfer control to the Servlet which is in the Same Webcontainer]
- Send Redirect Demo[Transfer control to different domain]
- Servlets : Send Redirect vs. Forward
- Page Redirection
- Filter Config
- Servlet Filter
- Servlet HttpSession
- Session Tracking
- Servlets Listeners
- Servlets Listeners
- ServletRequestAttributeListener Introduction
- ServletRequestAttributeListener Demo
- HttpSessionAttributeListener Introduction
- HttpSessionAttributeListener Demo
- ServletContextAttributeListener Introduction
- ServletContextAttributeListener Demo
- ServletContextListener Introduction
- ServletContextListener Demo
- HttpSessionListener Introduction
- HttpSessionListener Demo
- HttpSession Listener Demo [Count logged in Users]
- ServletRequestListener Introduction
- ServletRequestListener Demo
- HttpSessionBindinglistener Introduction
- HttpSessionBindinglistener Demo
- HttSessionActivationListener
- Servlets - Database Access
- MySQL Installation
- MySQL Workbench
- Oracle Database Server Installation
- Oracle Sql Developer Installation
- Oracle Sql Developer - Connect to the User
- JDBC|Servlets : BoneCP Connection Pooling - Oracle - Tomcat
- JDBC|Servlets : BoneCP Connection Pooling - Mysql- Tomcat
- JDBC|Servlets : BoneCP DataSource Oracle - Tomcat
- JDBC|Servlets : BoneCP DataSource Mysql - Tomcat
- JDBC|Servlets : Tomcat Connection Pooling - DBCP- Oracle
- JDBC|Servlets : Tomcat Connection Pooling - DBCP - Mysql
- JDBC|Servlets : Tomcat C3PO Connection Pooling - Mysql
- JDBC|Servlets : Tomcat C3PO Connection Pooling - Oracle
- Client-Server Model
- Eclipse
- Tomcat
- war file
- HTTP Methods and HTTP Status Codes
- Servlets Tutorial
- JDBC Tutorial
- JDBC Tutorial - Playlist [Part 1]
- JDBC Tutorial - Playlist
- Mysql Tutorial - Playlist
- JDBC : Steps to Connect to the Database and Demo - Playlist
- JDBC ResultSetMetadata - Playlist
- JDBC DatabaseMetadata - Playlist
- JDBC Statement - Playlist
- JDBC Connection - Playlist
- JDBC PreparedStatement - Playlist
- JDBC ResultSet - Playlist
- JDBC Create|Read|Update|Delete Records - Playlist
- JDBC Create|Drop Database - Playlist
- JDBC Create|Drop Table - Playlist
- JDBC Batch Processing - Playlist
- JDBC Scrollable ResultSet - Playlist
- JDBC Tutorial - Playlist [Part 2]
- JDBC DriverManager and JDBC Driver - Playlist
- Java Decompiler - Playlist
- JDBC Basics - Playlist
- JDBC Driver Types - Playlist
- JDBC Store and Retrieve Image - Playlist
- JDBC Store and Retrieve File - Playlist
- JDBC Transaction Management - Playlist
- JDBCRowSet - Playlist
- JDBC Mysql - Playlist
- JDBC Oracle - Playlist
- JDBC DataSource - Playlist
- JDBC DBCP DataSource - Playlist
- JDBC : Java Database Connection Pooling - Playlist
- Oracle Database & SqlDeveloper Tutorial - Playlist
- Bone CP Connection Pooling - Playlist
- DBCP Connection Pooling - Playlist
- C3PO Connection Pooling - Playlist
- JDBC Stored Procedure (Mysql) - Playlist
- JDBC Stored Procedure (Oracle) - Playlist
- Mysql & Oracle
- JDBC Basics
- JDBC Driver Types
- JDBC Driver Types
- Type 1 JDBC Driver : JDBC-ODBC Bridge Driver (Bridge Driver)
- Type 2 JDBC Driver: Native-API driver/Partly Java driver(Native Driver)
- Type 3 Driver : AllJava/Net-protocol driver or Network Protocol Driver(Middleware Driver)
- Type 4 Driver : All Java/Native-protocol driver or Thin Driver (Pure Java Driver)
- JDBC : Which Driver Should be used
- JDBC Statement & PreparedStatement
- JDBC ResultSet
- JDBC ResultSetMetadata
- JDBC DatabaseMetadata
- JDBC Create|Read|Update|Delete Records
- JDBC Batch Processing
- JDBC Transaction Management
- JDBC Store Image & File
- JDBCRowSet
- JDBC DataSource
- Java Database Connection Pooling
- JDBC : Java Database Connection Pooling [BoneCP vs DBPool vs C3PO]
- JDBC|Servlets : BoneCP Connection Pooling - Oracle - Tomcat
- JDBC|Servlets : BoneCP Connection Pooling - Mysql- Tomcat
- JDBC|Servlets : BoneCP DataSource Oracle - Tomcat
- JDBC|Servlets : BoneCP DataSource Mysql - Tomcat
- JDBC|Servlets : Tomcat Connection Pooling - DBCP- Oracle
- JDBC|Servlets : Tomcat Connection Pooling - DBCP - Mysql
- JDBC|Servlets : Tomcat C3PO Connection Pooling - Mysql
- JDBC|Servlets : Tomcat C3PO Connection Pooling - Oracle
- Object Pool Design Pattern - Introduction
- Object Pool Design Pattern - Implementation
- Connection Pooling [Example of Object Pool Design Pattern]
- Object Pool Design Pattern - KeyPoints
- JDBC Stored Procedure
- JDBC : CallableStatement Introduction.
- Mysql - Simple Stored Procedure
- Mysql - Stored Procedure with Input Parameter
- Mysql - Stored Procedure with multiple Input Parameters
- Mysql - Stored Procedure with Input and Output Parameters
- JDBC - CallableStatement with Input and Output Parameters(Mysql)
- JDBC - CallableStatement (Mysql)
- JDBC - CallableStatement with Input Parameter(Mysql)
- JDBC - CallableStatement Multiple In Out Parameters (Mysql)
- Oracle : Stored Procedure with Input and Output Parameters
- Oracle : Stored Procedure Cursor
- JDBC - CallableStatement In Out Parameters (Oracle)
- JDBC - CallableStatement Cursor(Oracle)
- JDBC - CallableStatement Cursor and multiple out param(Oracle)
- JDBC Tutorial
Friday 29 March 2019
Wednesday 27 March 2019
Sunday 24 March 2019
What is HibernateTransactionManager in Spring ?
Spring hibernate integration - Playlist
Click the below link to watch on Youtube:
https://www.youtube.com/watch?v=mdijsNXZx0U&list=PLmCsXDGbJHdih0tSOCtCCw_KOi52HuqH6
Spring 3 + hibernate 3 + Maven integration example
Sunday 17 March 2019
How to remove the Observer from the Observable in Java? | Observer and O...
Click the below link to watch on Youtube:
https://www.youtube.com/watch?v=fg712ek-c_Y
Example of Observer and Observable in Java? | Observer and Observable
What is the Observer and Observable in Java? | Observer and Observable - Playlist
Click the below link to watch on Youtube:
https://www.youtube.com/watch?v=o_Xbi8ZmkE8&list=PLmCsXDGbJHdh9VYKrQGTvM0wo2h8j3nzD
What is the Observer and Observable in Java? | Observer and Observable
Sunday 10 March 2019
Spring - How to get all records by calling the stored procedure using SimpleJdbcCall
Click here to watch on Youtube:
https://www.youtube.com/watch?v=ihdBllu4PSI&list=UUhwKlOVR041tngjerWxVccw
Spring - Calling a stored function using SimpleJdbcCall | Spring JDBC tutorial | Spring JDBC
Click here to watch on Youtube:
https://www.youtube.com/watch?v=5hf1kFQNCXs&list=UUhwKlOVR041tngjerWxVccw
Spring - Calling a stored procedure with SimpleJdbcCall (Explicitly declaring parameters)
Click here to watch on Youtube:
https://www.youtube.com/watch?v=uHXOTLzPdQ8&list=UUhwKlOVR041tngjerWxVccw
Spring - Calling a stored procedure with SimpleJdbcCall | Spring JDBC tutorial
Click here to watch on Youtube:
https://www.youtube.com/watch?v=VH9-d8XyWNY&list=UUhwKlOVR041tngjerWxVccw
Spring - Inserting data using SimpleJdbcInsert and MapSqlParameterSource | Spring JDBC tutorial
Click here to watch on Youtube:
https://www.youtube.com/watch?v=b3E_nXF6-qM&list=UUhwKlOVR041tngjerWxVccw
Spring - Inserting data using SimpleJdbcInsert and SqlParameterSource | Spring JDBC tutorial
Click here to watch on Youtube:
https://www.youtube.com/watch?v=SplB1Um2y3M&list=UUhwKlOVR041tngjerWxVccw
Wednesday 6 March 2019
Spring - Specifying columns for SimpleJdbcInsert | Spring JDBC tutorial | Spring JDBC
Click here to watch on Youtube:
https://www.youtube.com/watch?v=FNN0aWo9kWY&list=UUhwKlOVR041tngjerWxVccw
Spring - Inserting data using SimpleJdbcInsert | Spring JDBC tutorial | Spring JDBC
Click here to watch on Youtube:
https://www.youtube.com/watch?v=zCO1PqoXhKU&list=UUhwKlOVR041tngjerWxVccw
How to format the string using java.util.Formatter class - Playlist
Click here to watch on Youtube:
https://www.youtube.com/watch?v=qt89kDYMBGo&list=PLmCsXDGbJHdhKv54h0smpbiNuL9umvbUQ
See also:
All JavaEE Videos Playlist
All JavaEE Videos
All JAVA EE Links
Spring Tutorial
Servlets Tutorial
All Design Patterns Links
JDBC Tutorial
Java Collection Framework Tutorial
JAVA Tutorial
Kids Tutorial
Cooking Tutorial
https://www.youtube.com/watch?v=qt89kDYMBGo&list=PLmCsXDGbJHdhKv54h0smpbiNuL9umvbUQ
See also:
Tuesday 5 March 2019
How to use format(Locale l, String format, Object... args) method of java.util.Formatter class
Click here to watch on Youtube:
https://www.youtube.com/watch?v=ec2-YfmtPSk&list=UUhwKlOVR041tngjerWxVccw
FormatterDemo.java
Output:
Click the below link to download the code:
https://sites.google.com/site/javaspringram2019/java_spring_2019/FormatterDemo_format_locale.zip?attredirects=0&d=1
Github Link:
https://github.com/ramram43210/Java_Spring_2019/tree/master/Java_2019/FormatterDemo_format_locale
Bitbucket Link:
https://bitbucket.org/ramram43210/java_spring_2019/src/ef6f73b2185c28cb9cd0149bcd1e9fa0a5b2bdf3/Java_2019/FormatterDemo_format_locale/?at=master
See also:
All JavaEE Videos Playlist
All JavaEE Videos
All JAVA EE Links
Spring Tutorial
Servlets Tutorial
All Design Patterns Links
JDBC Tutorial
Java Collection Framework Tutorial
JAVA Tutorial
Kids Tutorial
Cooking Tutorial
https://www.youtube.com/watch?v=ec2-YfmtPSk&list=UUhwKlOVR041tngjerWxVccw
FormatterDemo.java
import java.util.Formatter;
import java.util.Locale;
/**
* public Formatter format(Locale l, String format, Object... args)
*
* Parameters:
*
* l - The locale to apply during formatting.
*
* format - A format string as described in Format string syntax.
*
* args - Arguments referenced by the format specifiers in the format
* string. If there are more arguments than format specifiers, the
* extra arguments are ignored. The maximum number of arguments is
* limited by the maximum dimension of a Java array as defined by The
* Java™ Virtual Machine Specification.
*
* Returns: This formatter
*
*/
public class FormatterDemo
{
public static void main(String[] args)
{
Formatter formatter = new Formatter();
/*
* In this example, the format specifiers, %s and %d are
* replaced with the arguments that follow the format string.
* %s is replaced by "Peter", %d is replaced by 10. %s
* specifies a string, and %d specifies an integer value. All
* other characters are simply used as-is.
*/
formatter.format(Locale.US, "%s age is %d", "Peter", 10);
System.out.println(formatter.out());
formatter.close();
}
}
import java.util.Locale;
/**
* public Formatter format(Locale l, String format, Object... args)
*
* Parameters:
*
* l - The locale to apply during formatting.
*
* format - A format string as described in Format string syntax.
*
* args - Arguments referenced by the format specifiers in the format
* string. If there are more arguments than format specifiers, the
* extra arguments are ignored. The maximum number of arguments is
* limited by the maximum dimension of a Java array as defined by The
* Java™ Virtual Machine Specification.
*
* Returns: This formatter
*
*/
public class FormatterDemo
{
public static void main(String[] args)
{
Formatter formatter = new Formatter();
/*
* In this example, the format specifiers, %s and %d are
* replaced with the arguments that follow the format string.
* %s is replaced by "Peter", %d is replaced by 10. %s
* specifies a string, and %d specifies an integer value. All
* other characters are simply used as-is.
*/
formatter.format(Locale.US, "%s age is %d", "Peter", 10);
System.out.println(formatter.out());
formatter.close();
}
}
Output:
Click the below link to download the code:
https://sites.google.com/site/javaspringram2019/java_spring_2019/FormatterDemo_format_locale.zip?attredirects=0&d=1
Github Link:
https://github.com/ramram43210/Java_Spring_2019/tree/master/Java_2019/FormatterDemo_format_locale
Bitbucket Link:
https://bitbucket.org/ramram43210/java_spring_2019/src/ef6f73b2185c28cb9cd0149bcd1e9fa0a5b2bdf3/Java_2019/FormatterDemo_format_locale/?at=master
See also:
How to format the string using java.util.Formatter class
Click here to watch on Youtube:
https://www.youtube.com/watch?v=qt89kDYMBGo&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge:
FormatterDemo.java
Output:
Refer:
https://docs.oracle.com/javase/8/docs/api/index.html?java/util/Formatter.html
Click the below link to download the code:
https://sites.google.com/site/javaspringram2019/java_spring_2019/FormatterDemo_Intro.zip?attredirects=0&d=1
Github Link:
https://github.com/ramram43210/Java_Spring_2019/tree/master/Java_2019/FormatterDemo_Intro
Bitbucket Link:
https://bitbucket.org/ramram43210/java_spring_2019/src/ef6f73b2185c28cb9cd0149bcd1e9fa0a5b2bdf3/Java_2019/FormatterDemo_Intro/?at=master
See also:
All JavaEE Videos Playlist
All JavaEE Videos
All JAVA EE Links
Spring Tutorial
Servlets Tutorial
All Design Patterns Links
JDBC Tutorial
Java Collection Framework Tutorial
JAVA Tutorial
Kids Tutorial
Cooking Tutorial
https://www.youtube.com/watch?v=qt89kDYMBGo&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge:
![]() |
How to format the string using java.util.Formatter class |
FormatterDemo.java
import java.util.Formatter;
/**
* public Formatter format(String format, Object... args)
*
* Parameters:
*
* format - A format string as described in Format string syntax.
*
* args - Arguments referenced by the format specifiers in the format
* string. If there are more arguments than format specifiers, the
* extra arguments are ignored. The maximum number of arguments is
* limited by the maximum dimension of a Java array as defined by The
* Java™ Virtual Machine Specification.
*
* Returns: This formatter
*
*/
public class FormatterDemo
{
public static void main(String[] args)
{
Formatter formatter = new Formatter();
/*
* In this example, the format specifiers, %s and %d are
* replaced with the arguments that follow the format string.
* %s is replaced by "Peter", %d is replaced by 10. %s
* specifies a string, and %d specifies an integer value. All
* other characters are simply used as-is.
*/
formatter.format("%s age is %d", "Peter", 10);
System.out.println(formatter.out());
formatter.close();
}
}
/**
* public Formatter format(String format, Object... args)
*
* Parameters:
*
* format - A format string as described in Format string syntax.
*
* args - Arguments referenced by the format specifiers in the format
* string. If there are more arguments than format specifiers, the
* extra arguments are ignored. The maximum number of arguments is
* limited by the maximum dimension of a Java array as defined by The
* Java™ Virtual Machine Specification.
*
* Returns: This formatter
*
*/
public class FormatterDemo
{
public static void main(String[] args)
{
Formatter formatter = new Formatter();
/*
* In this example, the format specifiers, %s and %d are
* replaced with the arguments that follow the format string.
* %s is replaced by "Peter", %d is replaced by 10. %s
* specifies a string, and %d specifies an integer value. All
* other characters are simply used as-is.
*/
formatter.format("%s age is %d", "Peter", 10);
System.out.println(formatter.out());
formatter.close();
}
}
Output:
Peter age is 10
Refer:
https://docs.oracle.com/javase/8/docs/api/index.html?java/util/Formatter.html
Click the below link to download the code:
https://sites.google.com/site/javaspringram2019/java_spring_2019/FormatterDemo_Intro.zip?attredirects=0&d=1
Github Link:
https://github.com/ramram43210/Java_Spring_2019/tree/master/Java_2019/FormatterDemo_Intro
Bitbucket Link:
https://bitbucket.org/ramram43210/java_spring_2019/src/ef6f73b2185c28cb9cd0149bcd1e9fa0a5b2bdf3/Java_2019/FormatterDemo_Intro/?at=master
See also:
Spring + JdbcTemplate + How to retrieve auto-generated keys
Click here to watch on Youtube:
https://www.youtube.com/watch?v=rbi3TkO0q5U&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge:
Employee.sql
pom.xml
EmployeeDAO.java
EmployeeDAOImpl.java
applicationContext.xml
App.java
Output:
Click the below link to download the code:
https://sites.google.com/site/javaspringram2019/java_spring_2019/SpringDemo_Retrive_auto_key.zip?attredirects=0&d=1
Github Link:
https://github.com/ramram43210/Java_Spring_2019/tree/master/Spring_2019/SpringDemo_Retrive_auto_key
Bitbucket Link:
https://bitbucket.org/ramram43210/java_spring_2019/src/8f8b5563b7f2921ed260908e4906103995d9c6cc/Spring_2019/SpringDemo_Retrive_auto_key/?at=master
See also:
All JavaEE Videos Playlist
All JavaEE Videos
All JAVA EE Links
Spring Tutorial
Servlets Tutorial
All Design Patterns Links
JDBC Tutorial
Java Collection Framework Tutorial
JAVA Tutorial
Kids Tutorial
Cooking Tutorial
https://www.youtube.com/watch?v=rbi3TkO0q5U&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge:
![]() |
Spring + JdbcTemplate + How to retrieve auto-generated keys |
Employee.sql
CREATE DATABASE org_db;
CREATE TABLE `employee` (
`EMPLOYEE_ID` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
`NAME` VARCHAR(100) NOT NULL,
`AGE` INT(10) NOT NULL,
`SALARY` INT(10) DEFAULT NULL,
PRIMARY KEY (`EMPLOYEE_ID`)
) ENGINE=INNODB AUTO_INCREMENT=4 DEFAULT CHARSET=utf8;
CREATE TABLE `employee` (
`EMPLOYEE_ID` INT(10) UNSIGNED NOT NULL AUTO_INCREMENT,
`NAME` VARCHAR(100) NOT NULL,
`AGE` INT(10) NOT NULL,
`SALARY` INT(10) DEFAULT NULL,
PRIMARY KEY (`EMPLOYEE_ID`)
) ENGINE=INNODB AUTO_INCREMENT=4 DEFAULT CHARSET=utf8;
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.ram.core</groupId>
<artifactId>SpringDemo</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>SpringDemo</name>
<url>http://maven.apache.org</url>
<properties>
<spring.version>5.0.5.RELEASE</spring.version>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Spring 5 dependencies -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- MySQL database driver -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.11</version>
</dependency>
</dependencies>
</project>
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.ram.core</groupId>
<artifactId>SpringDemo</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>SpringDemo</name>
<url>http://maven.apache.org</url>
<properties>
<spring.version>5.0.5.RELEASE</spring.version>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Spring 5 dependencies -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- MySQL database driver -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.11</version>
</dependency>
</dependencies>
</project>
EmployeeDAO.java
package com.ram.employee.dao;
public interface EmployeeDAO
{
public void retriveAutoGeneratedKey();
}
public interface EmployeeDAO
{
public void retriveAutoGeneratedKey();
}
EmployeeDAOImpl.java
package com.ram.employee.dao.impl;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import org.springframework.jdbc.core.PreparedStatementCreator;
import org.springframework.jdbc.core.support.JdbcDaoSupport;
import org.springframework.jdbc.support.GeneratedKeyHolder;
import org.springframework.jdbc.support.KeyHolder;
import com.ram.employee.dao.EmployeeDAO;
public class EmployeeDAOImpl
extends JdbcDaoSupport implements EmployeeDAO
{
public void retriveAutoGeneratedKey()
{
final String INSERT_SQL = "INSERT INTO EMPLOYEE "
+ "(NAME, AGE,SALARY) VALUES (?, ?, ?)";
KeyHolder keyHolder = new GeneratedKeyHolder();
getJdbcTemplate().update(new PreparedStatementCreator()
{
public PreparedStatement createPreparedStatement(
Connection connection) throws SQLException
{
PreparedStatement ps = connection.prepareStatement(
INSERT_SQL, new String[] { "id" });
ps.setString(1, "Rob");
ps.setInt(2, 12);
ps.setInt(3, 9090);
return ps;
}
}, keyHolder);
System.out.println(
"Auto generated Key is = " + keyHolder.getKey());
}
}
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import org.springframework.jdbc.core.PreparedStatementCreator;
import org.springframework.jdbc.core.support.JdbcDaoSupport;
import org.springframework.jdbc.support.GeneratedKeyHolder;
import org.springframework.jdbc.support.KeyHolder;
import com.ram.employee.dao.EmployeeDAO;
public class EmployeeDAOImpl
extends JdbcDaoSupport implements EmployeeDAO
{
public void retriveAutoGeneratedKey()
{
final String INSERT_SQL = "INSERT INTO EMPLOYEE "
+ "(NAME, AGE,SALARY) VALUES (?, ?, ?)";
KeyHolder keyHolder = new GeneratedKeyHolder();
getJdbcTemplate().update(new PreparedStatementCreator()
{
public PreparedStatement createPreparedStatement(
Connection connection) throws SQLException
{
PreparedStatement ps = connection.prepareStatement(
INSERT_SQL, new String[] { "id" });
ps.setString(1, "Rob");
ps.setInt(2, 12);
ps.setInt(3, 9090);
return ps;
}
}, keyHolder);
System.out.println(
"Auto generated Key is = " + keyHolder.getKey());
}
}
applicationContext.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.3.xsd">
<bean id="dataSource"
class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/org_db" />
<property name="username" value="root" />
<property name="password" value="root" />
</bean>
<bean id="employeeDAO" class="com.ram.employee.dao.impl.EmployeeDAOImpl">
<property name="dataSource" ref="dataSource" />
</bean>
</beans>
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.3.xsd">
<bean id="dataSource"
class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/org_db" />
<property name="username" value="root" />
<property name="password" value="root" />
</bean>
<bean id="employeeDAO" class="com.ram.employee.dao.impl.EmployeeDAOImpl">
<property name="dataSource" ref="dataSource" />
</bean>
</beans>
App.java
package com.ram.core;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.ram.employee.dao.EmployeeDAO;
public class App
{
public static void main(String[] args)
{
ApplicationContext context = new ClassPathXmlApplicationContext(
"applicationContext.xml");
EmployeeDAO employeeDAO = (EmployeeDAO) context
.getBean("employeeDAO");
employeeDAO.retriveAutoGeneratedKey();
}
}
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.ram.employee.dao.EmployeeDAO;
public class App
{
public static void main(String[] args)
{
ApplicationContext context = new ClassPathXmlApplicationContext(
"applicationContext.xml");
EmployeeDAO employeeDAO = (EmployeeDAO) context
.getBean("employeeDAO");
employeeDAO.retriveAutoGeneratedKey();
}
}
Output:
Feb 20, 2019 10:36:51 AM org.springframework.context.support.AbstractApplicationContext prepareRefresh
INFO: Refreshing org.springframework.context.support.ClassPathXmlApplicationContext@736e9adb: startup date [Wed Feb 20 10:36:51 IST 2019]; root of context hierarchy
Feb 20, 2019 10:36:52 AM org.springframework.beans.factory.xml.XmlBeanDefinitionReader loadBeanDefinitions
INFO: Loading XML bean definitions from class path resource [applicationContext.xml]
Loading class `com.mysql.jdbc.Driver'. This is deprecated. The new driver class is `com.mysql.cj.jdbc.Driver'. The driver is automatically registered via the SPI and manual loading of the driver class is generally unnecessary.
Feb 20, 2019 10:36:52 AM org.springframework.jdbc.datasource.DriverManagerDataSource setDriverClassName
INFO: Loaded JDBC driver: com.mysql.jdbc.Driver
Wed Feb 20 10:36:53 IST 2019 WARN: Establishing SSL connection without server's identity verification is not recommended. According to MySQL 5.5.45+, 5.6.26+ and 5.7.6+ requirements SSL connection must be established by default if explicit option isn't set. For compliance with existing applications not using SSL the verifyServerCertificate property is set to 'false'. You need either to explicitly disable SSL by setting useSSL=false, or set useSSL=true and provide truststore for server certificate verification.
Auto generated Key is = 10
INFO: Refreshing org.springframework.context.support.ClassPathXmlApplicationContext@736e9adb: startup date [Wed Feb 20 10:36:51 IST 2019]; root of context hierarchy
Feb 20, 2019 10:36:52 AM org.springframework.beans.factory.xml.XmlBeanDefinitionReader loadBeanDefinitions
INFO: Loading XML bean definitions from class path resource [applicationContext.xml]
Loading class `com.mysql.jdbc.Driver'. This is deprecated. The new driver class is `com.mysql.cj.jdbc.Driver'. The driver is automatically registered via the SPI and manual loading of the driver class is generally unnecessary.
Feb 20, 2019 10:36:52 AM org.springframework.jdbc.datasource.DriverManagerDataSource setDriverClassName
INFO: Loaded JDBC driver: com.mysql.jdbc.Driver
Wed Feb 20 10:36:53 IST 2019 WARN: Establishing SSL connection without server's identity verification is not recommended. According to MySQL 5.5.45+, 5.6.26+ and 5.7.6+ requirements SSL connection must be established by default if explicit option isn't set. For compliance with existing applications not using SSL the verifyServerCertificate property is set to 'false'. You need either to explicitly disable SSL by setting useSSL=false, or set useSSL=true and provide truststore for server certificate verification.
Auto generated Key is = 10
Click the below link to download the code:
https://sites.google.com/site/javaspringram2019/java_spring_2019/SpringDemo_Retrive_auto_key.zip?attredirects=0&d=1
Github Link:
https://github.com/ramram43210/Java_Spring_2019/tree/master/Spring_2019/SpringDemo_Retrive_auto_key
Bitbucket Link:
https://bitbucket.org/ramram43210/java_spring_2019/src/8f8b5563b7f2921ed260908e4906103995d9c6cc/Spring_2019/SpringDemo_Retrive_auto_key/?at=master
See also:
Spring + JdbcTemplate + How to create a table
Click here to watch on Youtube:
https://www.youtube.com/watch?v=S2EyOdg7dZM&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge:
Employee.sql
pom.xml
EmployeeDAO.java
EmployeeDAOImpl.java
applicationContext.xml
App.java
Output:
Click the below link to download the code:
https://sites.google.com/site/javaspringram2019/java_spring_2019/SpringDemo_create_table.zip?attredirects=0&d=1
Github Link:
https://github.com/ramram43210/Java_Spring_2019/tree/master/Spring_2019/SpringDemo_create_table
Bitbucket Link:
https://bitbucket.org/ramram43210/java_spring_2019/src/8f8b5563b7f2921ed260908e4906103995d9c6cc/Spring_2019/SpringDemo_create_table/?at=master
See also:
All JavaEE Videos Playlist
All JavaEE Videos
All JAVA EE Links
Spring Tutorial
Servlets Tutorial
All Design Patterns Links
JDBC Tutorial
Java Collection Framework Tutorial
JAVA Tutorial
Kids Tutorial
Cooking Tutorial
https://www.youtube.com/watch?v=S2EyOdg7dZM&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge:
![]() |
Spring + JdbcTemplate + How to create a table |
Employee.sql
CREATE DATABASE org_db;
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.ram.core</groupId>
<artifactId>SpringDemo</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>SpringDemo</name>
<url>http://maven.apache.org</url>
<properties>
<spring.version>5.0.5.RELEASE</spring.version>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Spring 5 dependencies -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- MySQL database driver -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.11</version>
</dependency>
</dependencies>
</project>
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.ram.core</groupId>
<artifactId>SpringDemo</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>SpringDemo</name>
<url>http://maven.apache.org</url>
<properties>
<spring.version>5.0.5.RELEASE</spring.version>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- Spring 5 dependencies -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- MySQL database driver -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.11</version>
</dependency>
</dependencies>
</project>
EmployeeDAO.java
package com.ram.employee.dao;
public interface EmployeeDAO
{
public void createTable();
}
public interface EmployeeDAO
{
public void createTable();
}
EmployeeDAOImpl.java
package com.ram.employee.dao.impl;
import org.springframework.jdbc.core.support.JdbcDaoSupport;
import com.ram.employee.dao.EmployeeDAO;
public class EmployeeDAOImpl
extends JdbcDaoSupport implements EmployeeDAO
{
public void createTable()
{
String sql = "create table Address (id integer, city varchar(100))";
getJdbcTemplate().execute(sql);
}
}
import org.springframework.jdbc.core.support.JdbcDaoSupport;
import com.ram.employee.dao.EmployeeDAO;
public class EmployeeDAOImpl
extends JdbcDaoSupport implements EmployeeDAO
{
public void createTable()
{
String sql = "create table Address (id integer, city varchar(100))";
getJdbcTemplate().execute(sql);
}
}
applicationContext.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.3.xsd">
<bean id="dataSource"
class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/org_db" />
<property name="username" value="root" />
<property name="password" value="root" />
</bean>
<bean id="employeeDAO" class="com.ram.employee.dao.impl.EmployeeDAOImpl">
<property name="dataSource" ref="dataSource" />
</bean>
</beans>
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.3.xsd">
<bean id="dataSource"
class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/org_db" />
<property name="username" value="root" />
<property name="password" value="root" />
</bean>
<bean id="employeeDAO" class="com.ram.employee.dao.impl.EmployeeDAOImpl">
<property name="dataSource" ref="dataSource" />
</bean>
</beans>
App.java
package com.ram.core;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.ram.employee.dao.EmployeeDAO;
public class App
{
public static void main(String[] args)
{
ApplicationContext context = new ClassPathXmlApplicationContext(
"applicationContext.xml");
EmployeeDAO employeeDAO = (EmployeeDAO) context
.getBean("employeeDAO");
employeeDAO.createTable();
System.out.println("Table is created successfully.");
}
}
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.ram.employee.dao.EmployeeDAO;
public class App
{
public static void main(String[] args)
{
ApplicationContext context = new ClassPathXmlApplicationContext(
"applicationContext.xml");
EmployeeDAO employeeDAO = (EmployeeDAO) context
.getBean("employeeDAO");
employeeDAO.createTable();
System.out.println("Table is created successfully.");
}
}
Output:
Feb 20, 2019 9:52:03 AM org.springframework.context.support.AbstractApplicationContext prepareRefresh
INFO: Refreshing org.springframework.context.support.ClassPathXmlApplicationContext@35d176f7: startup date [Wed Feb 20 09:52:03 IST 2019]; root of context hierarchy
Feb 20, 2019 9:52:04 AM org.springframework.beans.factory.xml.XmlBeanDefinitionReader loadBeanDefinitions
INFO: Loading XML bean definitions from class path resource [applicationContext.xml]
Loading class `com.mysql.jdbc.Driver'. This is deprecated. The new driver class is `com.mysql.cj.jdbc.Driver'. The driver is automatically registered via the SPI and manual loading of the driver class is generally unnecessary.
Feb 20, 2019 9:52:05 AM org.springframework.jdbc.datasource.DriverManagerDataSource setDriverClassName
INFO: Loaded JDBC driver: com.mysql.jdbc.Driver
Wed Feb 20 09:52:16 IST 2019 WARN: Establishing SSL connection without server's identity verification is not recommended. According to MySQL 5.5.45+, 5.6.26+ and 5.7.6+ requirements SSL connection must be established by default if explicit option isn't set. For compliance with existing applications not using SSL the verifyServerCertificate property is set to 'false'. You need either to explicitly disable SSL by setting useSSL=false, or set useSSL=true and provide truststore for server certificate verification.
Table is created successfully.
INFO: Refreshing org.springframework.context.support.ClassPathXmlApplicationContext@35d176f7: startup date [Wed Feb 20 09:52:03 IST 2019]; root of context hierarchy
Feb 20, 2019 9:52:04 AM org.springframework.beans.factory.xml.XmlBeanDefinitionReader loadBeanDefinitions
INFO: Loading XML bean definitions from class path resource [applicationContext.xml]
Loading class `com.mysql.jdbc.Driver'. This is deprecated. The new driver class is `com.mysql.cj.jdbc.Driver'. The driver is automatically registered via the SPI and manual loading of the driver class is generally unnecessary.
Feb 20, 2019 9:52:05 AM org.springframework.jdbc.datasource.DriverManagerDataSource setDriverClassName
INFO: Loaded JDBC driver: com.mysql.jdbc.Driver
Wed Feb 20 09:52:16 IST 2019 WARN: Establishing SSL connection without server's identity verification is not recommended. According to MySQL 5.5.45+, 5.6.26+ and 5.7.6+ requirements SSL connection must be established by default if explicit option isn't set. For compliance with existing applications not using SSL the verifyServerCertificate property is set to 'false'. You need either to explicitly disable SSL by setting useSSL=false, or set useSSL=true and provide truststore for server certificate verification.
Table is created successfully.
Click the below link to download the code:
https://sites.google.com/site/javaspringram2019/java_spring_2019/SpringDemo_create_table.zip?attredirects=0&d=1
Github Link:
https://github.com/ramram43210/Java_Spring_2019/tree/master/Spring_2019/SpringDemo_create_table
Bitbucket Link:
https://bitbucket.org/ramram43210/java_spring_2019/src/8f8b5563b7f2921ed260908e4906103995d9c6cc/Spring_2019/SpringDemo_create_table/?at=master
See also:
Subscribe to:
Posts (Atom)
Tutorials
- All Java EE Tutorial Links (4)
- All JAVA EE Tutorials (1)
- Apache Maven (5)
- Apache Maven Tutorial (1)
- Apache Server (2)
- Apache Server Tutorial (1)
- AWS Tutorial (1)
- Computer Tricks (32)
- Cooking (1)
- Design Patterns (330)
- Design Patterns Tutorial (1)
- Eclipse (5)
- Gradle (2)
- Gradle Tutorial (1)
- JAVA (2446)
- JAVA Basics (1522)
- Java Collection Framework (522)
- Java Collection Framework Tutorial (1)
- JAVA Tutorial (1)
- JDBC (117)
- JDBC Tutorial (1)
- JSON (25)
- JSON Tutorial (1)
- Kids Learning (16)
- Kids Learning Tutorial (1)
- Linux (8)
- Mysql (11)
- Oracle (10)
- Servlets (150)
- Servlets Tutorial (1)
- Spring (309)
- Spring Boot (173)
- Spring Boot Tutorial (1)
- Spring Tutorial (1)
- SQL (60)
- XML (9)