All Tutorials Links
Design Patterns
Servlets
JDBC
JAVA
Spring & Spring Boot & WebService & Database & AWS
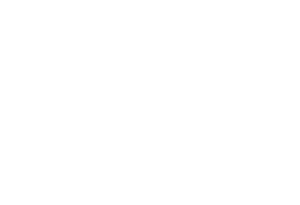
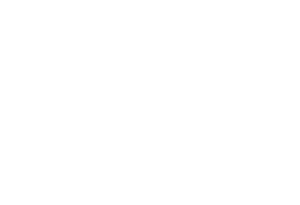
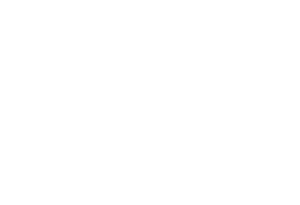
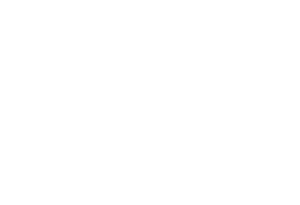
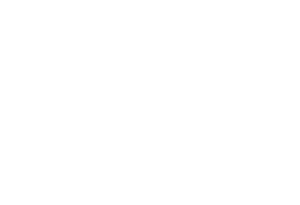
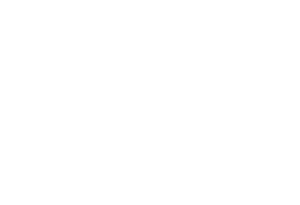
- All JavaEE Viedos Playlist
- All JavaEE Viedos
- All JAVA EE Links
- All Design Patterns Links
- Spring Tutorial
- Spring boot & JMS & Apache Kafka & Web Services
- Servlets Tutorial
- JDBC Tutorial
- JAVA Tutorial
- Java Collection Framework
- Apache Server Tutorial
- Apache Maven Tutorial
- JSON/XML/SQL/MongoDB Tutorials
- Computer Tutorial
- Kids Learning Tutorial
- Cooking Tutorial
- All Design Patterns Links
- Design Pattern - Playlists
- Design Pattern - Playlists - Part1
- Design Patterns - Introduction - Playlist
- J2EE Design Patterns - Playlist
- Creational Design patterns - Playlist
- Structural Design patterns - Playlist
- Behavioral Design patterns - Playlist
- Front Controller Design Pattern - Playlist
- Intercepting Filter Design Pattern - Playlist
- Business Delegate Design Pattern - Playlist
- Service Locator Design Pattern - Playlist
- Context Object Design Pattern - Playlist
- Data Access Object Design Pattern - Playlist
- Design Pattern - Playlists - Part2
- Object Pool Design Pattern - Playlist
- Singleton Design Pattern - Playlist
- Factory Design Pattern - Playlist
- Abstract Factory Design Pattern - Playlist
- Prototype Design pattern - Playlist
- Builder Design pattern - Playlist
- Iterator Design Pattern - Playlist
- Observer Design Pattern - Playlist
- Chain of Responsibility Design Pattern - Playlist
- Memento Design pattern - Playlist
- Design Pattern - Playlists - Part3
- Mediator Design pattern - Playlist
- Strategy Design pattern - Playlist
- Visitor Design pattern - Playlist
- State Design pattern - Playlist
- Command Design pattern - Playlist
- Interpreter Design pattern - Playlist
- Filter or Criteria Design Pattern - Playlist
- Composite Design Pattern - Playlist
- Proxy Design pattern - Playlist
- Flyweight Design pattern - Playlist
- Decorator Design pattern - Playlist
- Bridge Design pattern - Playlist
- Adapter Design pattern - Playlist
- Design Pattern - Playlists - Part4
- Base Design Pattern - Playlist
- Data Source Architectural Design Patterns - Playlist
- Object-Relational Metadata Mapping Design Patterns - Playlist
- Offline Concurrency Design Patterns - Playlist
- Domain Logic Design Patterns - Playlist
- Object-Relational Behavioral Design Patterns - Playlist
- Object-Relational Structural Design Patterns - Playlist
- Web Presentation Design Patterns - Playlist
- Distribution Design Patterns - Playlist
- Session State Design Patterns - Playlist
- Concurrency Design patterns - Playlist
- Design Pattern - Playlists - Part5
- Design Pattern - Playlists - Part1
- Design Patterns - Introduction
- Design Patterns
- Design patterns - catalog
- Enterprise Application Architecture Patterns
- Domain Logic Patterns
- Data Source Architectural Patterns
- Object Relational Behavioral Patterns
- Object-Relational Structural Patterns
- Object Relational Metadata Mapping Patterns
- Web Presentation Patterns
- Distribution Patterns
- Offline Concurrency Patterns
- Base Design Pattern
- Session State Design pattern
- Concurrency patterns
- J2EE patterns
- Creational Design patterns
- Structural design patterns
- Behavioral design patterns
- J2EE Design Patterns
- Creational Design patterns
- Creational Design patterns
- Object Pool Design Pattern
- Singleton Design Pattern
- Factory Design Pattern
- Abstract Factory Design Pattern
- Prototype Design Pattern
- Builder Design Pattern
- Builder Design Pattern - Introduction
- Builder Design pattern - Real Time Example [Meal Package]
- Builder Design pattern - Real Time Example [Animal Toys]
- Builder Design pattern - Real Time Example [Beverage]
- Builder Design Pattern - Class Diagram
- Builder Design Pattern - Sequence Diagram
- Builder Design Pattern - Implementation [Beverage]
- Builder Design Pattern - Implementation [Animal Toy]
- Builder Design Pattern - KeyPoints
- Behavioral design patterns
- Behavioral design patterns
- Iterator Design Pattern
- Observer Design Pattern
- Chain of Responsibility Design Pattern
- Chain of Responsibility Design Pattern - Introduction
- Chain of Responsibility Design Pattern - Real time Example
- Chain of Responsibility Design Pattern - Class and Sequence Diagram
- Chain of Responsibility Design Pattern - Implementation(One Receiver)
- Chain of Responsibility Design Pattern - Implementation(one or more Receiver)
- Chain of Responsibility Design Pattern - Key Points
- Momento Design pattern
- Template Design pattern or Template Method Design pattern
- Template Design pattern or Template Method Design pattern - Introduction
- Template Design pattern or Template Method Design pattern - Real Time Example [Coffee]
- Template Design pattern or Template Method Design pattern - Real Time Example [Car]
- Template Design pattern or Template Method Design pattern - Class Diagram
- Template Design pattern or Template Method Design pattern - Implementation [Building a House]
- Template Design pattern or Template Method Design pattern - Implementation [Coffee]
- Template Design pattern or Template Method Design pattern - KeyPoints
- Mediator Design pattern
- Mediator Design pattern - Introduction
- Mediator Design pattern - When to Use
- Mediator Design pattern - Real Time Example [Facebook]
- Mediator Design pattern - Real Time Example [ATC]
- Mediator Design pattern - Real Time Example [Chat Room]
- Mediator Design pattern - Class Diagram
- Mediator Design pattern - Implementation [Facebook]
- Mediator Design pattern - Implementation [Chat Room]
- Mediator Design pattern - KeyPoints
- Strategy Design pattern
- Strategy Design pattern - Introduction
- Strategy Design pattern - Real Time Example [Compress files]
- Strategy Design pattern - Real Time Example [Payment]
- Strategy Design pattern - Real Time Example [Travel]
- Strategy Design pattern - Real Time Example [Sorting]
- Strategy Design pattern - Real Time Example [Search]
- Strategy Design pattern - Class Diagram
- Strategy Design pattern - Sequence Diagram
- Strategy Design pattern - Implementation [Compress files]
- Strategy Design pattern - Implementation [Travel]
- Strategy Design pattern - Implementation [Payment]
- Strategy Design pattern - Implementation [Search]
- Strategy Design pattern - Implementation [Sort]
- Strategy Design pattern - KeyPoints
- Visitor Design pattern
- Visitor Design pattern - Introduction
- Visitor Design pattern - Real time Example [Kids]
- Visitor Design pattern - Real time Example [Products]
- Visitor Design pattern - Class Diagram
- Visitor Design pattern - Implementation [Kids]
- Visitor Design pattern - Implementation [Products]
- Visitor Design pattern - KeyPoints
- State Design pattern
- State Design pattern - Introduction
- State Design pattern - Real time example [ATM]
- State Design pattern - Real time example [TV Remote]
- State Design pattern - Real time example [Vending Machine]
- State Design pattern - Real time example [Project]
- State Design pattern - Class Diagram
- State Design pattern - Implementation [ATM]
- State Design pattern - Implementation [Vending Machine]
- State Design pattern - Implementation [Project]
- State Design pattern - Implementation [LED TV]
- State Design pattern - Keypoints
- Command Design pattern
- Command Design pattern - Introduction
- Command Design pattern - Real time example [Hotel]
- Command Design pattern - Real time example [Menu]
- Command Design pattern - Class Diagram
- Command Design pattern - Sequence Diagram
- Command Design pattern - Object Creation and flow
- Command Design pattern - Implementation [Menu]
- Command Design pattern - Key points
- Interpreter Design pattern
- Structural design patterns
- Structural design patterns
- Filter or Criteria Design Pattern
- Composite Design Pattern
- Proxy Design Pattern
- Proxy Design Pattern - Introduction
- Proxy Design pattern - Real Time Example[ATM]
- Proxy Design pattern - Real Time Example [Proxy Server]
- Proxy Design pattern - Class Diagram
- Proxy Design pattern - Sequence Diagram
- Proxy Design pattern - Implementation [Protection Proxy]
- Proxy Design pattern - Implementation [Remote Proxy]
- Proxy Design pattern - Implementation[Virtual Proxy]
- Proxy Design Pattern - Key Points
- Flyweight Design pattern
- Decorator Design pattern
- Decorator Design pattern - Introduction
- Decorator Design pattern - Real Time Example [Dosa]
- Decorator Design pattern - Real Time Example [Ice Cream]
- Decorator Design pattern - Real Time Example [Pizza]
- Decorator Design pattern - Real Time Example [Car]
- Decorator Design pattern - Class Diagram
- Decorator Design pattern - Sequence Diagram
- Decorator Design pattern - Implementation [Dosa]
- Decorator Design pattern - Implementation [Pizza]
- Decorator Design pattern - Implementation [Ice Cream]
- Decorator Design pattern - Implementation [Car]
- Decorator Design pattern - Implementation [Shape]
- Decorator Design pattern - Key Points
- Bridge Design pattern
- Bridge Design pattern - Introduction
- Bridge Design pattern - Real time example [Send Message]
- Bridge Design pattern - Real time example [Publish Message]
- Bridge Design pattern - Real time example [Shape]
- Bridge Design pattern - Real time example [TV]
- Bridge Design pattern - Class Diagram
- Bridge Design pattern - Implementation [Shape]
- Bridge Design pattern - Implementation [Send Message]
- Bridge Design pattern - Implementation [Publish Message]
- Bridge Design pattern - Implementation [LED TV]
- Bridge Design pattern - Keypoints
- Adapter Design Pattern
- Adapter Design pattern - Introduction
- Adapter Design Pattern - Real Time Exmaple(Translator)
- Adapter Design pattern - Real Time Example (Card Reader)
- Adapter Design pattern - Real Time Example (Mobile Charger)
- Adapter Design pattern - Real Time Example (Universal Adaptor)
- Adapter Design pattern - Real Time Example [JDBC Driver]
- Adapter Design pattern - Class diagram
- Adapter Design Pattern - Sequence diagram
- Adapter Design Pattern - Implementation [Language Translator]
- Adapter Pattern - Implementation [Object Adapter]
- Adapter Pattern - Implementation [Class Adapter]
- Adapter Design Pattern - Implementation [Mobile Charger]
- Adapter Design Pattern - Key Points
- Concurrency Design patterns
- Concurrency patterns
- Thread Pool Design Pattern
- Monitor or synchronization Design Pattern
- Lock Design Pattern
- Scheduler Design Pattern
- Read-write lock Design Pattern
- Messaging Design Pattern(MDP)
- Messaging Design Pattern(MDP) - Introduction
- Messaging Design Pattern(MDP) - Asynchronous messaging
- Messaging Design Pattern(MDP) - Two Way Messaging
- Messaging Design Pattern(MDP) - Implementation of Proxy
- Messaging Design Pattern(MDP)-Implementation of Adapter
- Messaging Design Pattern(MDP) - Implementation of Webservice
- Reactor Design Pattern
- Double-checked locking Design Pattern
- Offline Concurrency Patterns
- Session State Design pattern
- Distribution Patterns
- Base Design Pattern
- Base Design Pattern
- Gateway Design Pattern
- Layer Supertype Design Pattern
- Registry Design Pattern
- Money Design Pattern
- Plugin Design Pattern
- Special Case Design Pattern
- Separated Interface Design Pattern
- Service Stub Design Pattern
- Record Set Design Pattern
- Mapper Design Pattern
- Value Object Design Pattern
- Web Presentation Patterns
- Domain Logic Patterns
- Object Relational Structural Patterns
- Object-Relational Structural Patterns
- Identity Field Design Pattern
- Foreign Key Mapping Design Pattern
- Association Table Mapping Design Pattern
- Dependent Mapping Design Pattern
- Embedded Value Design Pattern
- Serialized LOB Design Pattern
- Single Table Inheritance Design Pattern
- Class Table Inheritance Design Pattern
- Concrete Table Inheritance Design Pattern
- Inheritance Mappers Design Pattern
- Object Relational Behavioral Patterns
- Object Relational MetadataMapping Patterns
- Data Source Architectural Patterns
- All Design Patterns Links
- Servlets Tutorial
- Servlets Tutorial - Playlists [Part 1]
- Servlets Tutorial - Playlist
- Tomcat - Playlist
- Servlets : WAR file - Playlist
- HTTP Methods and HTTP Status Codes - Playlist
- Client-Server Model - Playlist
- Servlet Application Creation using Eclipse - Playlist
- Servlets Basics - Playlist
- Servlets : Request and Response Headers - Playlist
- Servlets Form Data - Playlist
- Servlets : ServletConfig and ServletContext - Playlist
- Servlets : load-on-startup - Playlist
- Servlets : RequestDispatcher - Playlist
- Servlets : Send Redirect - Playlist
- Servlets Tutorial - Playlists [Part 2]
- Servlet Filter - Playlist
- Servlets : Filter Config - Playlist
- Servlets : HttpSession - Playlist
- Servlets : Cookies - Playlist
- Servlets : Attribute - Playlist
- Servlets : ServletRequestAttributeListener - Playlist
- Servlets : Listeners - Playlist
- Servlets : HttpSessionAttributeListener - Playlist
- Servlets : ServletContextAttributeListener - Playlist
- Servlets : ServletContextListener - Playlist
- Servlets : Upload File and Download File - Playlist
- Servlets : HttpSessionListener - Playlist
- Servlets : ServletRequestListener - Playlist
- Servlets : HttpSessionBindinglistener - Playlist
- Servlets : Session Tracking - Playlist
- Servlets : URL Rewriting - Playlist
- Servlets - Database Access - Playlist
- Servlets Basics - Part1
- Servlets Basics - Part2
- Servlets : Request and Response Headers
- Servlets Form Data
- ServletConfig and ServletContext
- Servlets : load-on-startup
- RequestDispatcher and Send Redirect
- RequestDispatcher Introduction
- Servlets : RequestDispatcher Example
- Servlets : Send Redirect Introduction
- Send Redirect Demo[Transfer control to the Servlet which is in the Same Webcontainer]
- Send Redirect Demo[Transfer control to different domain]
- Servlets : Send Redirect vs. Forward
- Page Redirection
- Filter Config
- Servlet Filter
- Servlet HttpSession
- Session Tracking
- Servlets Listeners
- Servlets Listeners
- ServletRequestAttributeListener Introduction
- ServletRequestAttributeListener Demo
- HttpSessionAttributeListener Introduction
- HttpSessionAttributeListener Demo
- ServletContextAttributeListener Introduction
- ServletContextAttributeListener Demo
- ServletContextListener Introduction
- ServletContextListener Demo
- HttpSessionListener Introduction
- HttpSessionListener Demo
- HttpSession Listener Demo [Count logged in Users]
- ServletRequestListener Introduction
- ServletRequestListener Demo
- HttpSessionBindinglistener Introduction
- HttpSessionBindinglistener Demo
- HttSessionActivationListener
- Servlets - Database Access
- MySQL Installation
- MySQL Workbench
- Oracle Database Server Installation
- Oracle Sql Developer Installation
- Oracle Sql Developer - Connect to the User
- JDBC|Servlets : BoneCP Connection Pooling - Oracle - Tomcat
- JDBC|Servlets : BoneCP Connection Pooling - Mysql- Tomcat
- JDBC|Servlets : BoneCP DataSource Oracle - Tomcat
- JDBC|Servlets : BoneCP DataSource Mysql - Tomcat
- JDBC|Servlets : Tomcat Connection Pooling - DBCP- Oracle
- JDBC|Servlets : Tomcat Connection Pooling - DBCP - Mysql
- JDBC|Servlets : Tomcat C3PO Connection Pooling - Mysql
- JDBC|Servlets : Tomcat C3PO Connection Pooling - Oracle
- Client-Server Model
- Eclipse
- Tomcat
- war file
- HTTP Methods and HTTP Status Codes
- Servlets Tutorial
- JDBC Tutorial
- JDBC Tutorial - Playlist [Part 1]
- JDBC Tutorial - Playlist
- Mysql Tutorial - Playlist
- JDBC : Steps to Connect to the Database and Demo - Playlist
- JDBC ResultSetMetadata - Playlist
- JDBC DatabaseMetadata - Playlist
- JDBC Statement - Playlist
- JDBC Connection - Playlist
- JDBC PreparedStatement - Playlist
- JDBC ResultSet - Playlist
- JDBC Create|Read|Update|Delete Records - Playlist
- JDBC Create|Drop Database - Playlist
- JDBC Create|Drop Table - Playlist
- JDBC Batch Processing - Playlist
- JDBC Scrollable ResultSet - Playlist
- JDBC Tutorial - Playlist [Part 2]
- JDBC DriverManager and JDBC Driver - Playlist
- Java Decompiler - Playlist
- JDBC Basics - Playlist
- JDBC Driver Types - Playlist
- JDBC Store and Retrieve Image - Playlist
- JDBC Store and Retrieve File - Playlist
- JDBC Transaction Management - Playlist
- JDBCRowSet - Playlist
- JDBC Mysql - Playlist
- JDBC Oracle - Playlist
- JDBC DataSource - Playlist
- JDBC DBCP DataSource - Playlist
- JDBC : Java Database Connection Pooling - Playlist
- Oracle Database & SqlDeveloper Tutorial - Playlist
- Bone CP Connection Pooling - Playlist
- DBCP Connection Pooling - Playlist
- C3PO Connection Pooling - Playlist
- JDBC Stored Procedure (Mysql) - Playlist
- JDBC Stored Procedure (Oracle) - Playlist
- Mysql & Oracle
- JDBC Basics
- JDBC Driver Types
- JDBC Driver Types
- Type 1 JDBC Driver : JDBC-ODBC Bridge Driver (Bridge Driver)
- Type 2 JDBC Driver: Native-API driver/Partly Java driver(Native Driver)
- Type 3 Driver : AllJava/Net-protocol driver or Network Protocol Driver(Middleware Driver)
- Type 4 Driver : All Java/Native-protocol driver or Thin Driver (Pure Java Driver)
- JDBC : Which Driver Should be used
- JDBC Statement & PreparedStatement
- JDBC ResultSet
- JDBC ResultSetMetadata
- JDBC DatabaseMetadata
- JDBC Create|Read|Update|Delete Records
- JDBC Batch Processing
- JDBC Transaction Management
- JDBC Store Image & File
- JDBCRowSet
- JDBC DataSource
- Java Database Connection Pooling
- JDBC : Java Database Connection Pooling [BoneCP vs DBPool vs C3PO]
- JDBC|Servlets : BoneCP Connection Pooling - Oracle - Tomcat
- JDBC|Servlets : BoneCP Connection Pooling - Mysql- Tomcat
- JDBC|Servlets : BoneCP DataSource Oracle - Tomcat
- JDBC|Servlets : BoneCP DataSource Mysql - Tomcat
- JDBC|Servlets : Tomcat Connection Pooling - DBCP- Oracle
- JDBC|Servlets : Tomcat Connection Pooling - DBCP - Mysql
- JDBC|Servlets : Tomcat C3PO Connection Pooling - Mysql
- JDBC|Servlets : Tomcat C3PO Connection Pooling - Oracle
- Object Pool Design Pattern - Introduction
- Object Pool Design Pattern - Implementation
- Connection Pooling [Example of Object Pool Design Pattern]
- Object Pool Design Pattern - KeyPoints
- JDBC Stored Procedure
- JDBC : CallableStatement Introduction.
- Mysql - Simple Stored Procedure
- Mysql - Stored Procedure with Input Parameter
- Mysql - Stored Procedure with multiple Input Parameters
- Mysql - Stored Procedure with Input and Output Parameters
- JDBC - CallableStatement with Input and Output Parameters(Mysql)
- JDBC - CallableStatement (Mysql)
- JDBC - CallableStatement with Input Parameter(Mysql)
- JDBC - CallableStatement Multiple In Out Parameters (Mysql)
- Oracle : Stored Procedure with Input and Output Parameters
- Oracle : Stored Procedure Cursor
- JDBC - CallableStatement In Out Parameters (Oracle)
- JDBC - CallableStatement Cursor(Oracle)
- JDBC - CallableStatement Cursor and multiple out param(Oracle)
- JDBC Tutorial
Thursday 27 August 2015
Java Tutorial : Java Inheritance IS-A and Has-A Relationship - Playlist
Java Tutorial : Java Inheritance IS-A Relationship - Playlist
Java Tutorial : Java Interface - Playlist
Java Tutorial : What is an Interface (Remote)
Click here to watch in Youtube :
https://www.youtube.com/watch?v=rLyF_27YtWw&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge
![]() |
Java Tutorial : What is an Interface (Remote) |
![]() |
Java Tutorial : What is an Interface (Remote) |
![]() |
Java Tutorial : What is an Interface (Remote) |
public class Person { private String name; private int age; public Person(String name, int age) { super(); this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } @Override public String toString() { return "Person [name=" + name + ", age=" + age + "]"; } public void switchOnTheTV() { System.out.println(name+" is calling switchOn method of Remote "); Remote remote = new LEDTVRemote(); remote.switchOn(); } public void switchOffTheTV() { System.out.println(name+" is calling switchOff method of Remote "); Remote remote = new LEDTVRemote(); remote.switchOff(); } }
/** * An interface is a group of related methods with empty bodies. */ public interface Remote { void switchOn(); void switchOff(); }
public class LEDTVRemote implements Remote { @Override public void switchOn() { System.out.println("switchOn method of LEDTVRemote is called" + " and Remote is calling switchOnTV method of LED TV "); LedTV ledtv = new LedTV("106 Cm",true); ledtv.switchOnTV(); } @Override public void switchOff() { System.out.println("switchOff method of LEDTVRemote is called" + " and Remote is calling switchOffTV method of LED TV "); LedTV ledtv = new LedTV("106 Cm",true); ledtv.switchOffTV(); } }
public class LedTV { private String displaySize; private boolean isSmartTv; public LedTV(String displaySize, boolean isSmartTv) { super(); this.displaySize = displaySize; this.isSmartTv = isSmartTv; } public String getDisplaySize() { return displaySize; } public void setDisplaySize(String displaySize) { this.displaySize = displaySize; } public boolean isSmartTv() { return isSmartTv; } public void setSmartTv(boolean isSmartTv) { this.isSmartTv = isSmartTv; } public void switchOnTV() { System.out.println("switchOnTV method of LEDTV is called."); System.out.println("LED TV is Switched on."); } public void switchOffTV() { System.out.println("switchOffTV method of LEDTV is called."); System.out.println("LED TV is Switched off."); } }
public class InterfaceDemo { public static void main(String[] args) { Person peter = new Person("Peter",28); peter.switchOnTheTV(); System.out.println("-----------------------------------------------"); peter.switchOffTheTV(); } }
Peter is calling switchOn method of Remote switchOn method of LEDTVRemote is called and Remote is calling switchOnTV method of LED TV switchOnTV method of LEDTV is called. LED TV is Switched on. ----------------------------------------------- Peter is calling switchOff method of Remote switchOff method of LEDTVRemote is called and Remote is calling switchOffTV method of LED TV switchOffTV method of LEDTV is called. LED TV is Switched off.
https://sites.google.com/site/javaee4321/java/InterfaceDemoLedApp.zip?attredirects=0&d=1
See also:
Java Tutorial : What is an Interface (Switch)
Click here to watch in Youtube :
https://www.youtube.com/watch?v=x8SqfAWYE9E&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge
![]() |
Java Tutorial : What is an Interface (Switch) |
![]() |
Java Tutorial : What is an Interface (Switch) |
![]() |
Java Tutorial : What is an Interface (Switch) |
public class Person { private String name; private int age; public Person(String name, int age) { super(); this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } @Override public String toString() { return "Person [name=" + name + ", age=" + age + "]"; } public void switchOnTheBulb() { Switch bulbswitch = new LEDBulbSwitch(); bulbswitch.switchOn(); } public void switchOffTheBulb() { Switch bulbswitch = new LEDBulbSwitch(); bulbswitch.switchOff(); } }
/** * An interface is a group of related methods with empty bodies. */ public interface Switch { void switchOn(); void switchOff(); }
public class LEDBulbSwitch implements Switch { @Override public void switchOn() { LedBulb ledbulb = new LedBulb("'A' shape","2.3 inch"); ledbulb.switchOnTheLedBulb(); } @Override public void switchOff() { LedBulb ledbulb = new LedBulb("'A' shape","2.3 inch"); ledbulb.switchOffTheLedBulb(); } }
public class LedBulb { private String shape; private String diameter; public LedBulb(String shape, String diameter) { super(); this.shape = shape; this.diameter = diameter; } public String getShape() { return shape; } public void setShape(String shape) { this.shape = shape; } public String getDiameter() { return diameter; } public void setDiameter(String diameter) { this.diameter = diameter; } public void switchOnTheLedBulb() { System.out.println(shape +" Led Bulb is switched on"); } public void switchOffTheLedBulb() { System.out.println(shape +" Led Bulb is switched off"); } }
public class InterfaceDemo { public static void main(String[] args) { Person peter = new Person("Peter",28); peter.switchOnTheBulb(); peter.switchOffTheBulb(); } }
'A' shape Led Bulb is switched on 'A' shape Led Bulb is switched off
https://sites.google.com/site/javaee4321/java/InterfaceDemoSwitchApp.zip?attredirects=0&d=1
See also:
Tuesday 25 August 2015
Java Tutorial : Inheritance Has A Relationship (Person)
Click here to watch in Youtube :
https://www.youtube.com/watch?v=p_4U_LVMbSk&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge
![]() |
Java Tutorial : Inheritance Has A Relationship (Person) |
![]() |
Java Tutorial : Inheritance Has A Relationship (Person) |
![]() |
Java Tutorial : Inheritance Has A Relationship (Person) |
![]() |
Java Tutorial : Inheritance Has A Relationship (Person) |
![]() |
Java Tutorial : Inheritance Has A Relationship (Person) |
public class Person
{ private String name; private int age; public Person(String name, int age) { super(); this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } @Override public String toString() { return "Person [name=" + name + ", age=" + age + "]"; } }
public class Address { private String street; private String city; private String state; private String zip; public Address(String street, String city, String state, String zip) { super(); this.street = street; this.city = city; this.state = state; this.zip = zip; } public String getStreet() { return street; } public void setStreet(String street) { this.street = street; } public String getCity() { return city; } public void setCity(String city) { this.city = city; } public String getState() { return state; } public void setState(String state) { this.state = state; } public String getZip() { return zip; } public void setZip(String zip) { this.zip = zip; } @Override public String toString() { return "Address [street=" + street + ", city=" + city + ", state=" + state + ", zip=" + zip + "]"; } }
/** * * Employee class extends Person class means Employee IS-A Person. * * Employee extends Person and thus inherits all methods and properties from * Person (except final and static). * * Employee can also define all its specific functionality. */ public class Employee extends Person { private String departmentName; private int employeeId; /* * Employee HAS-A Address */ private Address address; public Employee(String name, int age, String departmentName, int employeeId, Address address) { super(name, age); this.departmentName = departmentName; this.employeeId = employeeId; this.address = address; } public String getDepartmentName() { return departmentName; } public void setDepartmentName(String departmentName) { this.departmentName = departmentName; } public int getEmployeeId() { return employeeId; } public void setEmployeeId(int employeeId) { this.employeeId = employeeId; } public Address getAddress() { return address; } public void setAddress(Address address) { this.address = address; } @Override public String toString() { return "Employee [departmentName=" + departmentName + ", employeeId=" + employeeId + ", address=" + address + ", getName()=" + getName() + ", getAge()=" + getAge() + "]"; } }
/** * * Student class extends Person class means Student IS-A Person. * * Student extends Person and thus inherits all methods and properties from * Person (except final and static). * * Student can also define all its specific functionality. */ public class Student extends Person { private double grade; private int rollNo; /* * Student HAS-A Address */ private Address address; public Student(String name, int age, double grade, int rollNo, Address address) { super(name, age); this.grade = grade; this.rollNo = rollNo; this.address = address; } public double getGrade() { return grade; } public void setGrade(double grade) { this.grade = grade; } public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public Address getAddress() { return address; } public void setAddress(Address address) { this.address = address; } @Override public String toString() { return "Student [grade=" + grade + ", rollNo=" + rollNo + ", address=" + address + ", getName()=" + getName() + ", getAge()=" + getAge() + "]"; } }
public class RelationshipDemo { public static void main(String[] args) { Address peterAddress = new Address("13th Cross", "Bangalore", "Karnataka", "560001"); Employee peter = new Employee("Peter", 33, "Income Tax Department", 350, peterAddress); System.out.println("Employee Peter Details : "); System.out.println(peter); System.out.println("----------------------------------------------"); Address johnAddress = new Address("17th Cross", "Chennai", "TamilNadu", "600100"); Student john = new Student("John", 33, 3.5, 2, johnAddress); System.out.println("Student john Details : "); System.out.println(john); } }Output
Employee Peter Details : Employee [departmentName=Income Tax Department, employeeId=350, address=Address [street=13th Cross, city=Bangalore, state=Karnataka, zip=560001], getName()=Peter, getAge()=33] ---------------------------------------------- Student john Details : Student [grade=3.5, rollNo=2, address=Address [street=17th Cross, city=Chennai, state=TamilNadu, zip=600100], getName()=John, getAge()=33]To Download InheritanceDemo-HAS-A-PersonApp Project Click the below link
https://sites.google.com/site/javaee4321/java/InheritanceDemo-HAS-A-PersonApp.zip?attredirects=0&d=1
See also:
Monday 24 August 2015
Java Tutorial : Inheritance Has A Relationship (Car)
Click here to watch in Youtube :
https://www.youtube.com/watch?v=lQlu0kel2x8&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge
![]() |
Java Tutorial : Inheritance Has A Relationship (Car) |
![]() |
Java Tutorial : Inheritance Has A Relationship (Car) |
public class Car { private String color; private int maxSpeed; public Car(String color, int maxSpeed) { super(); this.color = color; this.maxSpeed = maxSpeed; } public String getColor() { return color; } public void setColor(String color) { this.color = color; } public int getMaxSpeed() { return maxSpeed; } public void setMaxSpeed(int maxSpeed) { this.maxSpeed = maxSpeed; } public void carInfo() { System.out.println("Car Color= " + color + ", Max Speed= " + maxSpeed); } }
public class Engine { public void start() { System.out.println("Engine Started:"); } public void stop() { System.out.println("Engine Stopped:"); } }
/** * * MarutiSwift is specific type of Car which extends Car class means MarutiSwift * IS-A Car. * * MarutiSwift extends Car and thus inherits all properties and methods from Car * (except final and static). * * MarutiSwift can also define all its specific functionality. */ public class MarutiSwift extends Car { /* * MarutiSwift HAS-A Engine */ private Engine engine; public MarutiSwift(String color, int maxSpeed, Engine engine) { super(color, maxSpeed); this.engine = engine; } public void startMarutiSwift() { engine.start(); } }RelationshipDemo.java
/** * RelationsDemo class is making object of MarutiSwift class and initialized it. * Though MarutiSwift class does not have setColor(), setMaxSpeed() and carInfo() * methods still we can use it due to IS-A relationship of MarutiSwift class with Car * class. */ public class RelationshipDemo { public static void main(String[] args) { Engine engine = new Engine(); MarutiSwift marutiSwift = new MarutiSwift("Red", 200, engine); marutiSwift.carInfo(); marutiSwift.startMarutiSwift(); } }
Car Color= Red, Max Speed= 200 Engine Started:To Download InheritanceDemo-HAS-A-CarApp Project Click the below link
https://sites.google.com/site/javaee4321/java/InheritanceDemo-HAS-A-CarApp.zip?attredirects=0&d=1
See also:
Friday 21 August 2015
Java Tutorial : Inheritance IS A Relationship Introduction
Click here to watch in Youtube :
https://www.youtube.com/watch?v=Rz4VuCNeUNQ&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge
![]() |
Java Tutorial : Inheritance IS A Relationship Introduction |
![]() |
Java Tutorial : Inheritance IS A Relationship Introduction |
Thursday 20 August 2015
Java Tutorial : Inheritance IS A Relationship
Click here to watch in Youtube :
https://www.youtube.com/watch?v=1axqHoHqyIU&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge
![]() |
Java Tutorial : Inheritance IS A Relationship |
![]() |
Java Tutorial : Inheritance IS A Relationship |
Java Tutorial : Inheritance Types
Click here to watch in Youtube :
https://www.youtube.com/watch?v=4JGA20J3kls&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge
![]() |
Java Tutorial : Inheritance Types |
![]() |
Java Tutorial : Inheritance Types |
Wednesday 19 August 2015
Java Tutorial : Inheritance IS-A Relationship Animal (Implements)
Click here to watch in Youtube :
https://www.youtube.com/watch?v=oXtnBDbGo5k&list=UUhwKlOVR041tngjerWxVccw
Click the below Image to Enlarge
![]() |
Java Tutorial : Inheritance IS-A Relationship Animal (Implements) |
![]() |
Java Tutorial : Inheritance IS-A Relationship Animal (Implements) |
![]() |
Java Tutorial : Inheritance IS-A Relationship Animal (Implements) |
/** * Animal is the Super Interface of Mammal,Parrot and Dove classes */ interface Animal { } /** * Mammal is the subclass of Animal Interface. */ class Mammal implements Animal { } /** * Dog is the subclass of both Mammal class and Animal Interface. */ class Dog extends Mammal { } /** * Elephant is the subclass of both Mammal class and Animal Interface. */ class Elephant extends Mammal { } /** * Tiger is the subclass of both Mammal class and Animal Interface. */ class Tiger extends Mammal { } /** * Parrot is the subclass of Animal Interface. */ class Parrot implements Animal { } /** * Dove is the subclass of Animal Interface. */ class Dove implements Animal { } public class AnimalDemo { public static void main(String args[]) { Mammal mammal = new Mammal(); Dog dog = new Dog(); Elephant elephant = new Elephant(); Tiger tiger = new Tiger(); System.out.print("Mammal IS-A Animal : "); System.out.println(mammal instanceof Animal); System.out.println(); System.out.print("Dog IS-A Mammal : "); System.out.println(dog instanceof Mammal); System.out.print("Dog IS-A Animal : "); System.out.println(dog instanceof Animal); System.out.println(); System.out.print("Elephant IS-A Mammal : "); System.out.println(elephant instanceof Mammal); System.out.print("Elephant IS-A Animal : "); System.out.println(elephant instanceof Animal); System.out.println(); System.out.print("Tiger IS-A Mammal : "); System.out.println(tiger instanceof Mammal); System.out.print("Tiger IS-A Animal : "); System.out.println(tiger instanceof Animal); System.out.println(); Parrot parrot = new Parrot(); Dove dove = new Dove(); System.out.print("Parrot IS-A Animal : "); System.out.println(parrot instanceof Animal); System.out.println(); System.out.print("Dove IS-A Animal : "); System.out.println(dove instanceof Animal); } }
Mammal IS-A Animal : true
Dog IS-A Mammal : true Dog IS-A Animal : true Elephant IS-A Mammal : true Elephant IS-A Animal : true Tiger IS-A Mammal : true Tiger IS-A Animal : true Parrot IS-A Animal : true Dove IS-A Animal : true
https://sites.google.com/site/javaee4321/java/InheritanceDemo-IS-A-Animal-ImplementsApp.zip?attredirects=0&d=1
See also:
Subscribe to:
Posts (Atom)
Tutorials
- All Java EE Tutorial Links (4)
- All JAVA EE Tutorials (1)
- Apache Maven (5)
- Apache Maven Tutorial (1)
- Apache Server (2)
- Apache Server Tutorial (1)
- AWS Tutorial (1)
- Computer Tricks (32)
- Cooking (1)
- Design Patterns (330)
- Design Patterns Tutorial (1)
- Eclipse (5)
- Gradle (2)
- Gradle Tutorial (1)
- JAVA (2446)
- JAVA Basics (1522)
- Java Collection Framework (522)
- Java Collection Framework Tutorial (1)
- JAVA Tutorial (1)
- JDBC (117)
- JDBC Tutorial (1)
- JSON (25)
- JSON Tutorial (1)
- Kids Learning (16)
- Kids Learning Tutorial (1)
- Linux (8)
- Mysql (11)
- Oracle (10)
- Servlets (150)
- Servlets Tutorial (1)
- Spring (309)
- Spring Boot (173)
- Spring Boot Tutorial (1)
- Spring Tutorial (1)
- SQL (60)
- XML (9)